Web accessibility is often overlooked, but it's crucial for ensuring that all users, regardless of ability, can use your application. In this post, I'll share practical strategies for building more accessible React applications.
Why Accessibility Matters
Beyond being the right thing to do, accessible websites:
- Reach a wider audience (approximately 15% of the world's population has some form of disability)
- Improve SEO and overall user experience
- Help meet legal requirements in many jurisdictions
- Force you to write cleaner, more semantic code
Semantic HTML: The Foundation
Always start with proper HTML elements. For example, use <button>
for interactive elements that users will click, not a styled <div>
:
// Bad
<div onClick={handleClick} className="button">Click Me</div>
// Good
<button onClick={handleClick}>Click Me</button>
Form Accessibility
Forms are often troublesome for users with disabilities. Here are some tips:
- Always use labels and associate them with inputs
- Provide clear error messages
- Use ARIA attributes when necessary
- Ensure keyboard navigation works properly
// Accessible form example
<form>
<div>
<label htmlFor="name">Name</label>
<input
id="name"
type="text"
aria-required="true"
aria-invalid={errors.name ? "true" : "false"}
/>
{errors.name && (
<span role="alert" className="error">
{errors.name}
</span>
)}
</div>
</form>
Focus Management
Proper focus management is crucial for keyboard users. Here are some guidelines:
- Ensure all interactive elements are keyboard accessible
- Maintain a logical tab order
- Make focus indicators visible
- Use
focus-visible
for better user experience
Testing for Accessibility
Use these tools to check your application:
- Lighthouse in Chrome DevTools
- axe DevTools browser extension
- WAVE Web Accessibility Evaluation Tool
- Screen readers (VoiceOver on Mac, NVDA on Windows)
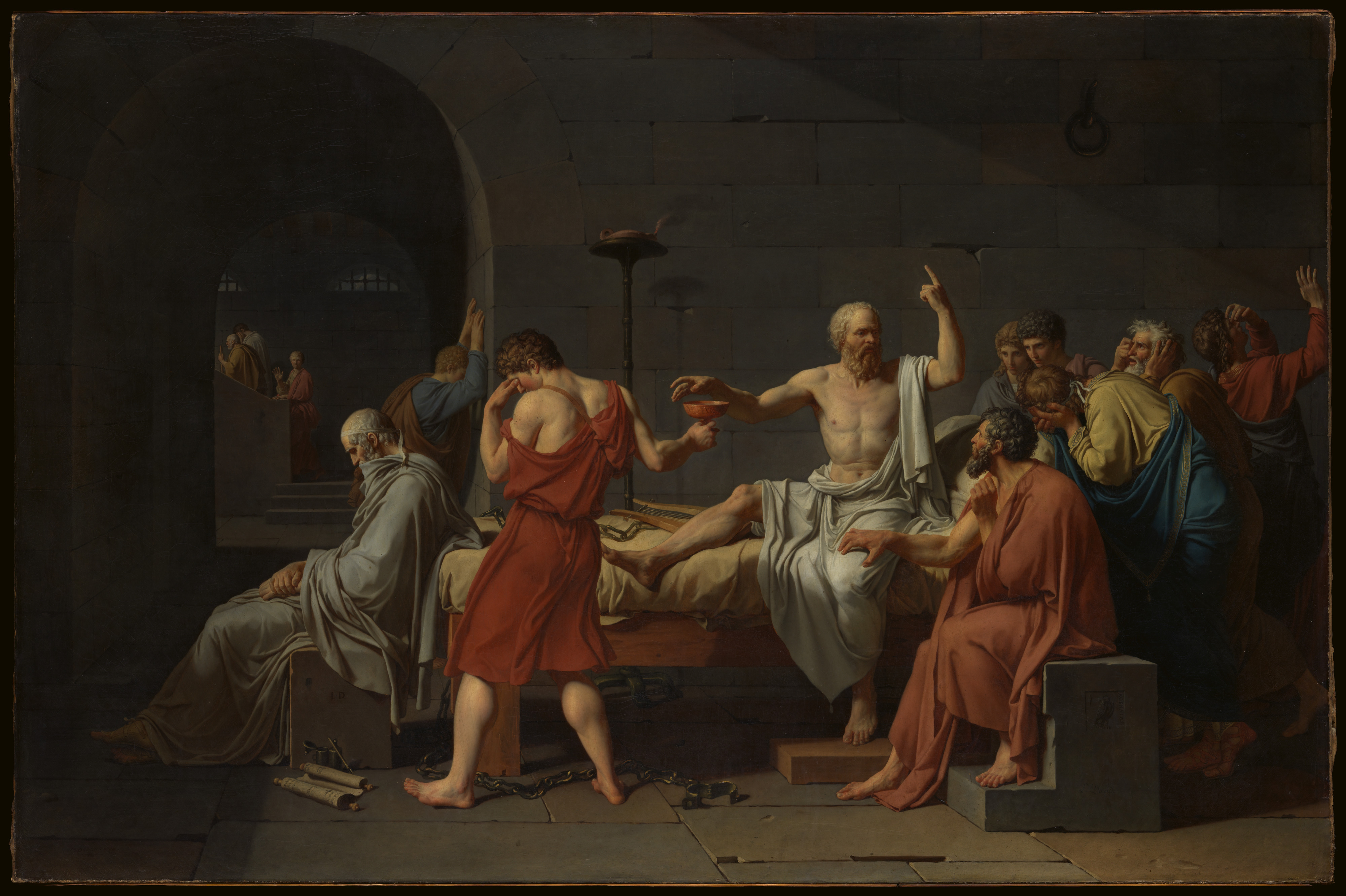
Remember that automated tools catch only about 30% of issues, so manual testing is essential.
Resources to Learn More
- Web Content Accessibility Guidelines (WCAG)
- React Accessibility Docs
The A11Y Project
Building accessible applications takes practice, but it becomes second nature over time. Start incorporating these practices into your workflow, and you'll be creating more inclusive experiences for all users.
Comments